Understanding the Structure of a Jetpack Compose Project in Android
Jetpack Compose is a modern UI toolkit for building native Android applications using a declarative approach. As it gains popularity, many developers are transitioning from XML-based layouts to Compose. Understanding the structure of a Jetpack Compose project is crucial for building efficient and maintainable applications. This article will walk you through the typical structure of a Jetpack Compose project, highlighting key components and best practices.
1. Overview of Jetpack Compose
Jetpack Compose simplifies UI development by using a declarative paradigm, where the UI is defined in Kotlin code rather than XML. This approach allows developers to describe how the UI should look at any given time and let Compose handle the updates as the state changes. The structure of a Jetpack Compose project is organized around composable functions, which are the building blocks of the UI.
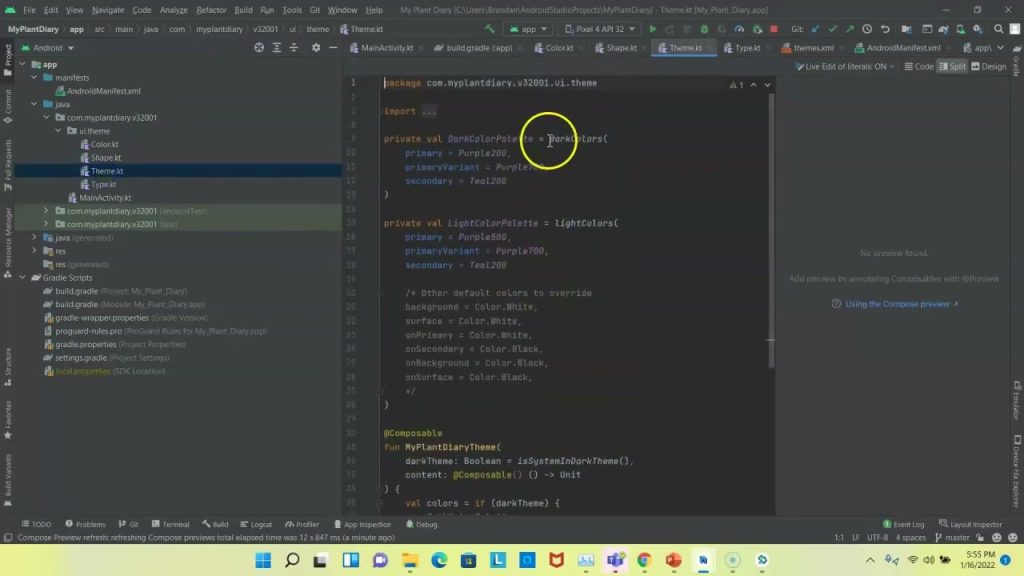
2. Project Structure
A Jetpack Compose project follows a similar directory structure to a traditional Android project, with some key differences to accommodate Compose-specific elements.
2.1 Project Root
At the root of your project, you’ll find standard Android project files:
build.gradle
(Project-Level): This file defines the build configurations for the entire project, including repositories, classpaths, and plugins.settings.gradle
: This file specifies the modules that make up your project.
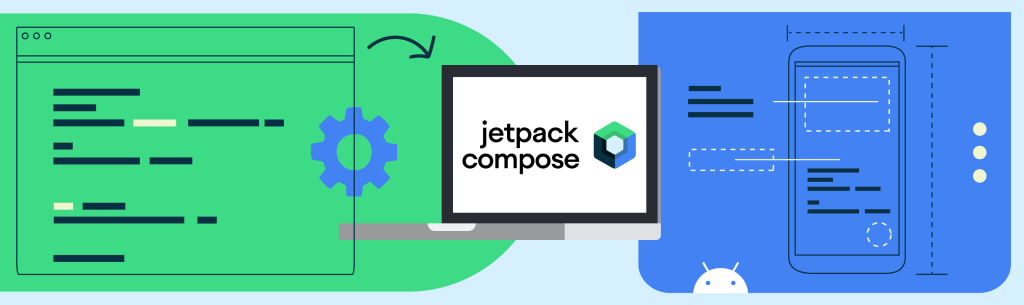
2.2 App Module
The main module of your project (usually named app
) contains most of your app’s source code and resources. Within this module, the directory structure is as follows:
src/main/java/com/yourpackage/
: This directory contains all the Kotlin source files, including your composable functions, state management classes, and other logic.src/main/res/
: This directory holds the app’s resources, such as drawable assets, strings, and colors. Even though Jetpack Compose reduces the reliance on XML, this directory still plays an essential role in resource management.src/main/AndroidManifest.xml
: This file declares essential information about your app to the Android system, such as activities, permissions, and services.
3. Key Components of a Jetpack Compose Project
3.1 Composable Functions
Composable functions are the core building blocks of Jetpack Compose. Each composable function is a self-contained unit of UI that can be combined with other composables to build complex interfaces.
- Composable Annotation (
@Composable
): Every composable function is annotated with@Composable
. This tells the Compose compiler that this function will produce UI elements. - Example
Greeting
is a simple composable function that takes a string as input and displays it as text.
@Composable
fun Greeting(name: String) {
Text(text = "Hello, $name!")
}
3.2 State Management
State management is integral to Jetpack Compose, as it determines how the UI responds to changes over time. Compose offers several ways to manage state, such as remember
, State
, MutableState
, and ViewModel
.
remember
: Theremember
function helps Compose remember the state across recompositions. It is used to store simple state that doesn’t need to survive configuration changes.State
andMutableState
: These classes are used to create and manage state variables that can trigger recompositions when they change.- Example
count
is a state variable that updates the UI whenever it changes.
@Composable
fun Counter() {
var count by remember { mutableStateOf(0) }
Column {
Text(text = "Count: $count")
Button(onClick = { count++ }) {
Text("Increment")
}
}
}
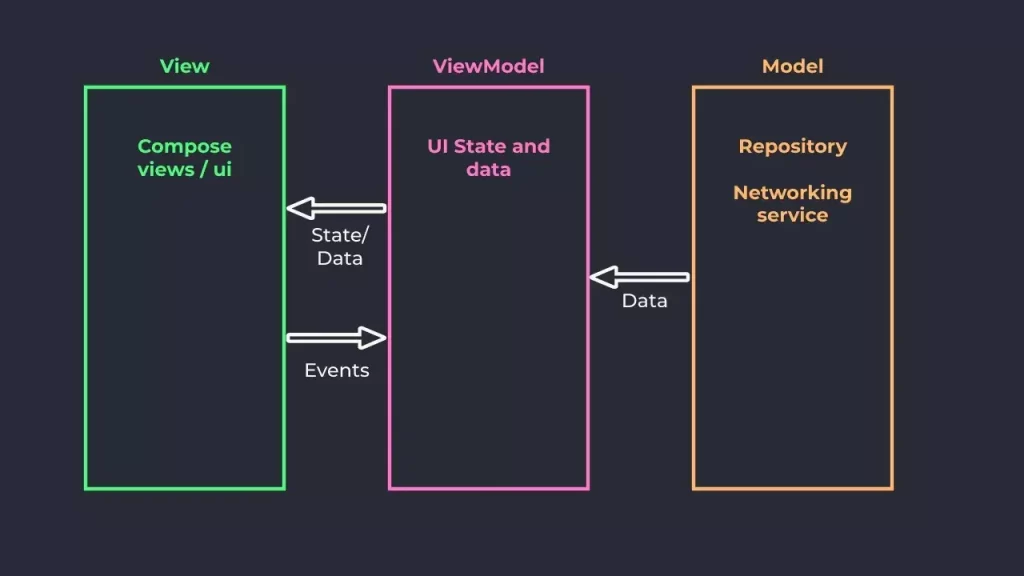
3.3 Themes and Styles
In Jetpack Compose, theming is done through the MaterialTheme
composable, which provides a consistent look and feel across the app. Themes are defined using color schemes, typography, and shapes.
- Material Design: Jetpack Compose includes built-in support for Material Design components, making it easy to create visually appealing UIs that adhere to design guidelines.
- Custom Themes: You can create custom themes by overriding the
MaterialTheme
properties.
@Composable
fun MyAppTheme(content: @Composable () -> Unit) {
MaterialTheme(
colors = myColors,
typography = myTypography,
shapes = myShapes,
content = content
)
}
3.4 Navigation
Navigation in Jetpack Compose is handled by the NavController
and NavHost
components from the androidx.navigation.compose
package. These components manage the navigation stack and allow you to define navigation routes between different composables.
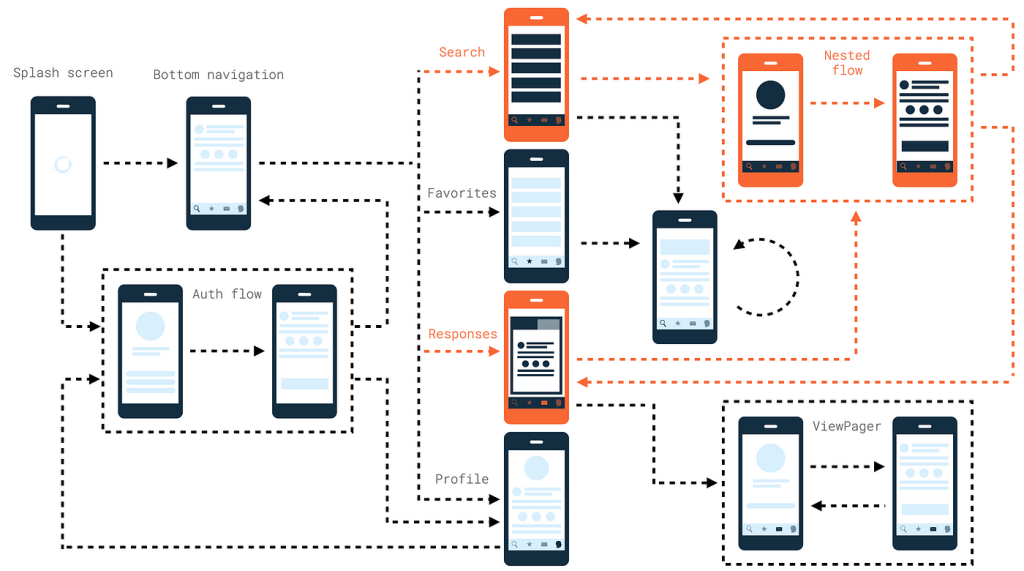
- NavHost: Defines the navigation graph, specifying the start destination and the routes between composables.
- NavController: Manages the navigation stack and handles navigation actions like navigating to a new screen or going back.
- Example:This sets up navigation between a
HomeScreen
and aDetailsScreen
.
@Composable
fun MyAppNavHost(navController: NavHostController) {
NavHost(navController = navController, startDestination = "home") {
composable("home") { HomeScreen(navController) }
composable("details") { DetailsScreen(navController) }
}
}
3.5 ViewModel Integration
Jetpack Compose integrates seamlessly with the Android ViewModel
, allowing you to manage UI-related data in a lifecycle-aware manner.
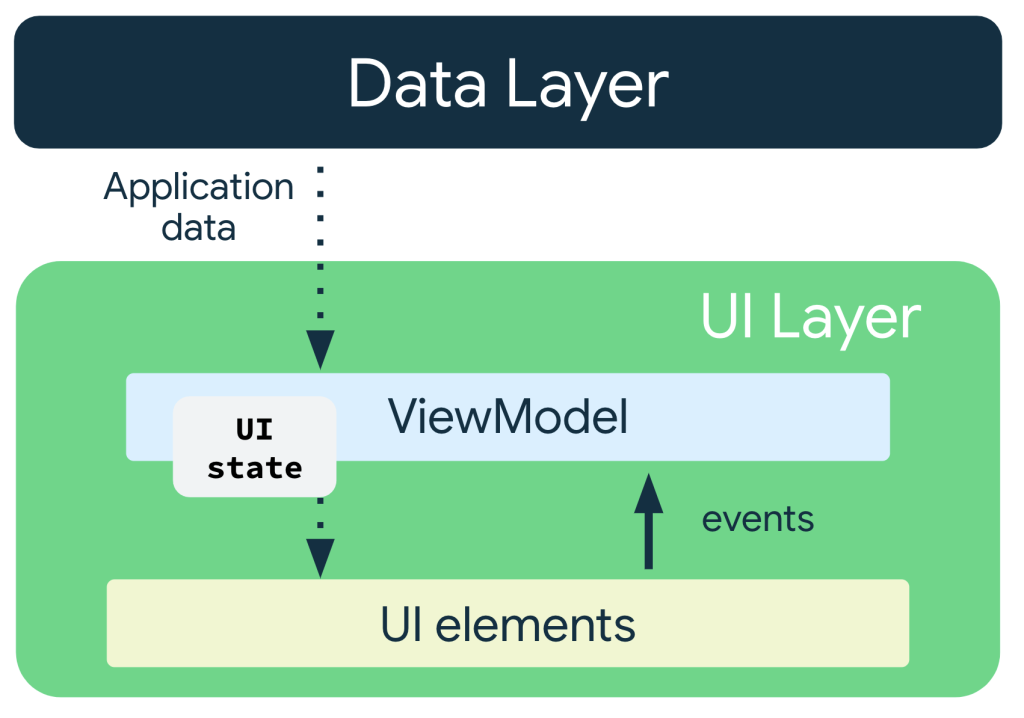
- ViewModel and LiveData: Use
ViewModel
to store and manage UI-related data. In Compose,LiveData
orStateFlow
can be observed to trigger UI updates. - Example:
ViewModel
and update the UI accordingly.
@Composable
fun MyScreen(viewModel: MyViewModel = viewModel()) {
val data by viewModel.data.observeAsState()
data?.let {
// Render your UI here
}
}
4. Testing in Jetpack Compose
Testing is a crucial aspect of any development process. Jetpack Compose offers a set of testing APIs that enable you to write UI tests for composable functions.
- Compose Test Rule: The
ComposeTestRule
provides a set of tools for testing composables, such as setting the content and interacting with UI elements. - Writing Tests: You can use functions like
onNodeWithText()
oronNodeWithTag()
to locate and interact with UI elements in your tests. - Example:This test checks that the
Greeting
composable displays the correct text.
@Test
fun testGreeting() {
composeTestRule.setContent {
Greeting(name = "World")
}
composeTestRule.onNodeWithText("Hello, World!").assertExists()
}
5. Best Practices in Jetpack Compose Project Structure
To ensure maintainability and scalability, adhere to the following best practices in your Jetpack Compose project:
- Modularize Your Code: Break down your project into smaller modules if it becomes too large. This helps with organization and reusability.
- Keep UI and Logic Separate: Although Compose allows embedding logic in UI code, it’s essential to maintain a clear separation between UI (composables) and business logic (ViewModel).
- Consistent Theming: Use a consistent theme throughout your app by leveraging
MaterialTheme
and custom themes. This improves the user experience and makes the app visually cohesive. - Utilize State Management Properly: Manage state effectively using Compose’s state management tools, and avoid excessive recompositions by structuring your composables efficiently.
Conclusion
Jetpack Compose brings a fresh approach to Android UI development, with a project structure that encourages modularity, reusability, and clear state management. Understanding the structure of a Jetpack Compose project and adhering to best practices will help you build scalable, maintainable, and efficient Android applications. As Compose continues to evolve, mastering these concepts will become increasingly important for Android developers looking to stay ahead in the rapidly changing mobile development landscape.