Understanding Activities in Android: A Comprehensive Guide
In Android development, an Activity represents one of the most fundamental building blocks of an application. An Activity is the entry point for interacting with a user and serves as a single screen with a user interface. This article provides an in-depth understanding of what an Android Activity is, its lifecycle, types, and how to implement and manage activities in Android development.
What is an Activity in Android?
An Activity in Android is a crucial component of an Android app, representing a single, focused thing that a user can do. Typically, each Activity corresponds to a screen in the app. Activities handle the visual user interface and interact with the users to take input and respond to events.
For example, in a messaging app, you might have multiple activities:
- One for viewing a list of messages.
- Another for composing a new message.
Each activity is typically associated with a user interface defined in XML files (though Jetpack Compose offers an alternative UI approach). The core role of an Activity is to facilitate interaction with the user and maintain the UI.
Key Responsibilities of an Activity
An Android Activity performs several key roles within an application, including:
- Displaying UI elements: Activities display layouts and interact with various UI components like buttons, text fields, and images.
- Handling User Interactions: Activities capture user inputs and respond to them by triggering appropriate actions, such as opening a new activity or updating a UI element.
- Coordinating with Other Components: An Activity may start another activity, communicate with a service, or interact with a content provider.
- Managing the Activity Lifecycle: An activity must manage its state, respond to lifecycle events, and be aware of context changes (like screen rotation or app backgrounding).
Activity Lifecycle
The Activity Lifecycle refers to the stages an Activity goes through during its existence—from creation to destruction. Understanding the lifecycle is essential for ensuring that your app functions smoothly and performs optimally. The lifecycle includes various callbacks that allow developers to manage transitions between different states:
Lifecycle Callbacks:
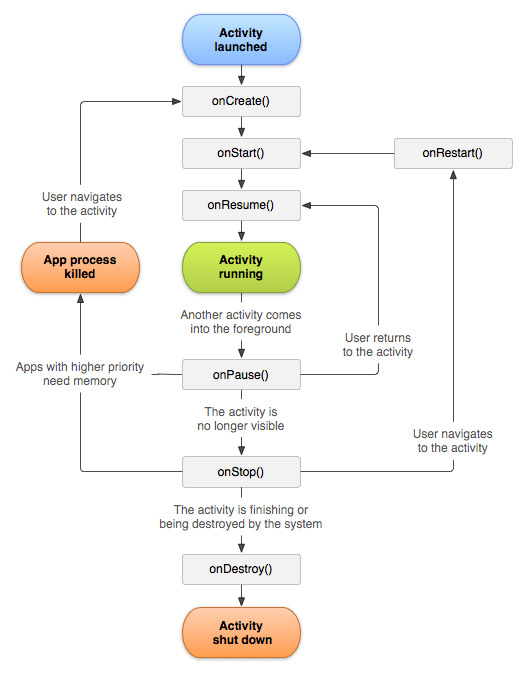
onCreate()
:
- Called when the activity is first created.
- You typically initialize the UI and any necessary data here.
- Example:
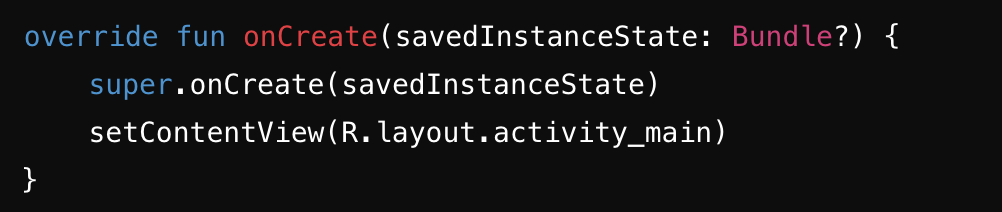
onStart()
:
- Called when the activity becomes visible to the user but not yet in the foreground.
onResume()
:
- Called when the activity is ready for interaction with the user.
- This is where you typically start animations or tasks that need to run as the activity is in the foreground.
onPause()
:
- Called when the activity is partially obscured, such as when another activity comes in front.
- This is where you save UI state and pause animations or data updates.
onStop()
:
- Called when the activity is no longer visible to the user. It’s still alive but in the background.
onDestroy()
:
- Called when the activity is about to be destroyed either because the user is exiting the activity or the system is temporarily destroying it to save space.
onRestart()
:
- Called after
onStop()
, just before the activity starts again.
Types of Activities
Android applications can have various types of activities, each serving a different purpose:
Main Activity:
The main activity is typically the first screen users see when they launch the app. It’s specified as the app’s entry point in the AndroidManifest.xml
file using an intent filter for the “main” action and “launcher” category:
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
Sub-Activities:
Sub-activities are activities that are called from other activities to perform specific tasks, like showing details or handling settings. They are typically launched using an Intent
object:
val intent = Intent(this, DetailsActivity::class.java)
startActivity(intent)
Dialog Activity:
This is a special type of activity that presents itself as a dialog. It doesn’t occupy the full screen and is useful for tasks like user confirmations or warnings.
<activity
android:name=".DialogActivity"
android:theme="@android:style/Theme.Dialog">
</activity>
SingleTop and SingleTask Activities:
These are special activity launch modes that determine how the activity behaves in the task stack. For instance, singleTop
activities are reused if they already exist at the top of the stack, while singleTask
activities create a new task if necessary.
Intents: Navigating Between Activities
Intents are how Android apps navigate between activities and pass data. Intents can be used to launch a new activity or perform an action, such as sending an email or opening a web page.
- Explicit Intent: Used to launch a specific activity within the app.
Implicit Intent: Used when you want to perform an action, but don’t specify which app should handle it. The system will prompt the user to choose an app.
Intents can also pass data between activities via putExtra() and getExtras() methods.
Conclusion
Activities are a core component of Android applications, responsible for presenting the user interface and managing user interactions. By understanding the activity lifecycle, navigating between activities using intents, handling configuration changes, and managing resources effectively, you can build robust and user-friendly Android apps. As Android continues to evolve, activities remain a key concept, even as components like fragments and Jetpack Compose change how we think about UI development.