Structure of an Android Project
When developing an Android application, it’s essential to have a clear understanding of the project structure. Android project are designed with a specific organization that helps manage code, resources, and dependencies efficiently. In this article, we’ll dive deep into the Android project structure, explain its key components, and show how they work together to create a well-organized, maintainable application.
1. Overview of the Android Project Structure
An Android project is typically organized into multiple directories, files, and configuration settings, each serving a distinct purpose. The structure is modular and follows a standard that separates code from resources, helping developers manage projects efficiently across different screens, languages, and Android versions.
At the root level, the structure of a typical Android project includes:
- The Gradle Scripts
- The App Module
- The Manifest File
- The Java/Kotlin Source Code
- The Resource Directory (res)
2. Gradle Scripts
Gradle is the build system used in Android projects. It automates the compilation, testing, and packaging of your application. Each project typically contains two main Gradle files:
2.1 build.gradle (Project-level)
This file defines the configuration that applies to the entire project. It includes settings such as the repository for dependencies (e.g., Maven, Google), and Gradle plugins (like the Android plugin) that apply to all modules in the project.
2.2 build.gradle (Module-level)
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath "com.android.tools.build:gradle:7.0.2"
}
}
The module-level build.gradle
file contains configurations specific to the app module. It defines application-level settings, such as compile SDK versions, minimum and target SDK versions, dependencies, and flavor configurations.
android {
compileSdkVersion 31
defaultConfig {
applicationId "com.example.myapp"
minSdkVersion 21
targetSdkVersion 31
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
}
}
}
In addition to these, you will have other Gradle files like gradle.properties
for environment variables and settings.gradle
for specifying included modules.
3. App Module
The app module contains all the code and resources for the Android application. It is usually the primary module for a simple app, but in complex applications, you can have multiple modules for separation of concerns.
The src/
directory in the app module contains the following subdirectories:
main/
: This is where most of the project’s logic and resources reside. It contains subdirectories for Java/Kotlin code, XML layouts, drawable resources, and more.androidTest/
andtest/
: These directories contain unit and instrumentation tests, which help test the app’s functionality.
4. The Manifest File
The Android AndroidManifest.xml
file is a critical part of the Android project structure. It provides essential information about the app to the Android system, such as package name, activities, permissions, and app components like services or receivers.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapp">
<application
android:allowBackup="true"
android:label="@string/app_name"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Permissions: The manifest is where you declare permissions, such as internet access or reading the user’s contacts.
Activities: Every activity in the app must be registered in the manifest.
Application Metadata: This is where you set app-wide settings like themes or permissions.
5. Java/Kotlin Source Code
Inside the src/main/java/
or src/main/kotlin/
directory, you will find all your app’s source code. By default, the structure is organized based on the package name you chose during project creation. It might look like this:
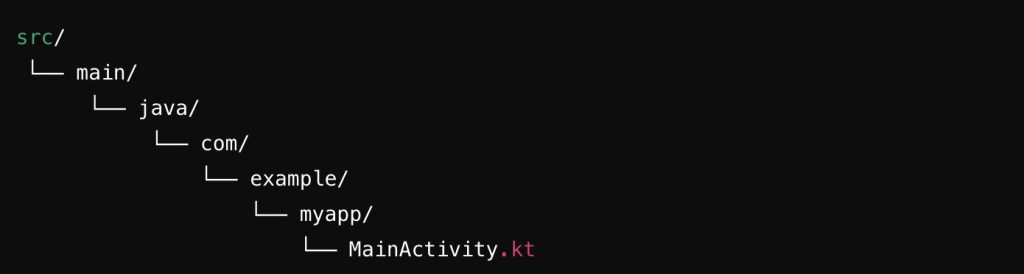
Activities: The core components of Android apps that manage user interaction, like MainActivity.kt
.Fragments: Reusable components representing parts of the UI.ViewModel: Classes that manage UI-related data in a lifecycle-conscious way.Repositories/Services: Data-handling classes that interact with databases, APIs, or other data sources.
6. The Resources Directory (res)
The res/
directory is a vital part of the Android project structure. It holds all the non-code resources that the app uses, such as layouts, strings, images, and styles.
6.1 Layouts
Layouts are XML files that define the visual structure of your app. Each screen of the app is defined by a layout file, which is typically located in the res/layout/
directory.
- Example layout file (
activity_main.xml
):
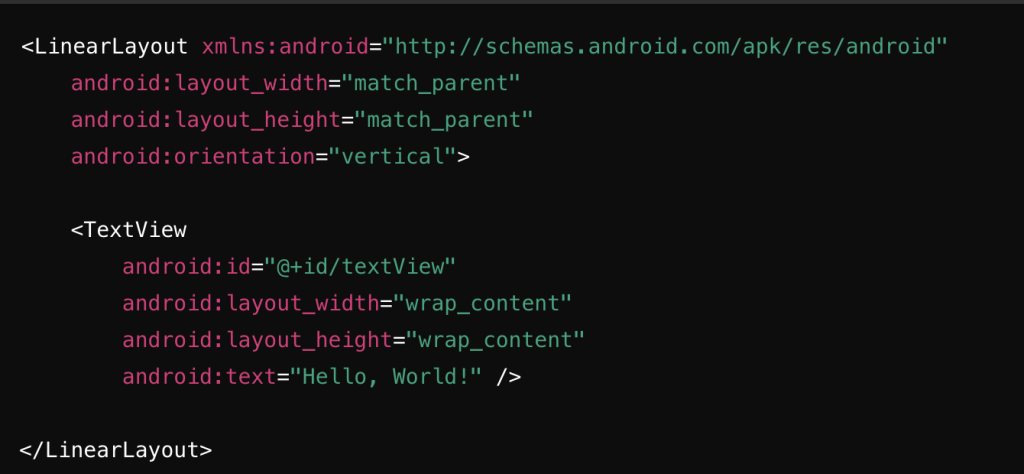
6.2 Drawables
The res/drawable/
directory holds image files and XML graphics such as shapes, gradients, and selectors. Drawable resources are used for UI elements like icons, backgrounds, or other graphic components.
6.3 Values
The res/values/
directory contains XML files that store various resources like strings, dimensions, and styles, allowing you to centralize and reuse these resources throughout the app.
strings.xml
: Stores the app’s string resources, which can be referenced from other XML files or code.colors.xml
: Holds color definitions that can be applied to UI elements.styles.xml
: Defines themes and styles used to apply consistent design elements across the app.
6.4 MipMap
The res/mipmap/
directory is used to store app icons at different resolutions. This ensures that your app icon looks sharp on devices with different screen densities.
6.5 Other Resource Directories
res/raw/
: A directory for storing raw files such as audio, videos, or other media files.
res/menu/
: Contains XML files that define the menu structure for activities or fragments.
res/anim/
and res/animator/
: Used for defining animations or transitions between UI elements.
7. Testing Directory
Testing is an essential part of Android development, and the Android project structure supports both unit and UI testing.
src/androidTest/
: This directory contains instrumentation tests, which run on physical or virtual Android devices.src/test/
: This directory contains local unit tests that can run on your development machine’s JVM.
Both directories use testing libraries like JUnit or Espresso to ensure that the app functions as expected.
8. Libraries and Dependencies
The libs/
directory holds external libraries or .jar files that the project uses. However, most modern Android projects manage dependencies through Gradle’s dependency management system by adding them to the build.gradle
file:

9. App Module Build Variants
In large Android projects, you may want to build different versions of your app, such as debug
and release
builds. Android’s build system supports product flavors and build types to customize these variants.
- Product Flavors: Allow you to create different versions of your app with variations like free vs. paid versions.
- Build Types: Specify how the app is built, for example, enabling or disabling code obfuscation and debugging tools.
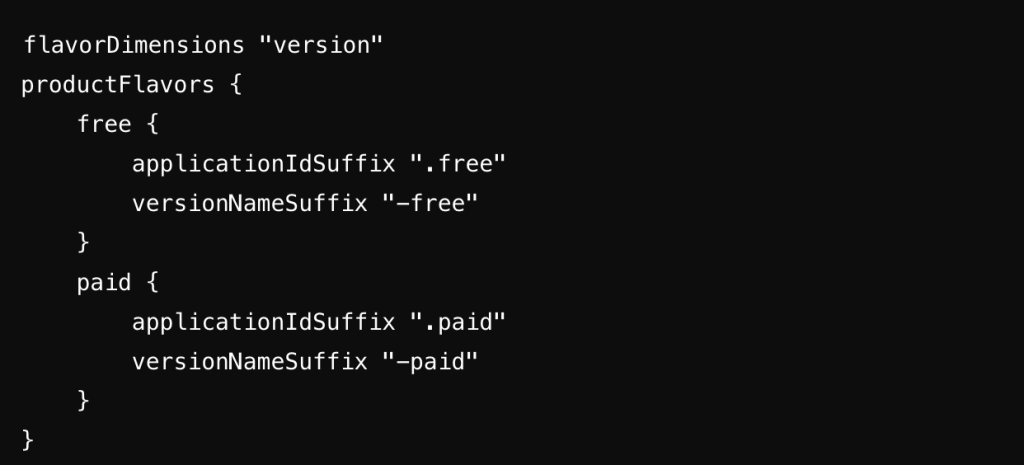
Conclusion
Understanding the structure of an Android project is key to effective development. Android’s project structure is designed to separate code, resources, and configuration files in a way that encourages scalability and maintainability. Each component has a specific role, from the build.gradle
files to the res/
directory and the manifest file. Mastering this structure will help you navigate and manage your projects with confidence as you build robust and efficient Android applications.